概要
Lightning Web ComponentのDatatable(lightning-datatable)は、簡単にテーブル形式でデータを表示することができるコンポーネントです。Datatableでは、標準でサポートしている型(※)がありますが、カスタマイズしたい場合にはLightningDatatableを拡張してCustom Typeを定義することができます。Custom Typeにより例えば行削除ボタンや画像、カスタムのテキストや数値のセルを実装することができます。
※Summer’23時点
action
boolean
button
button-icon
currency
date
date-local
email
location
number
percent
phone
text (default)
url
Custom Data Typeについて
Custom Data Typeを使用するには、LightningDatatableを拡張したコンポーネントを作成する必要があります。
今回は、サンプルである一定の条件を満たした際にボタンを表示/非表示を切り替えるCustom Typeを定義してみました。
サンプル
取引先のデータテーブルでカスタムデータタイプで表示条件をつけたボタンを作ってみました。
一番右の列のRelated listは取引先に関連するケースが存在する場合にのみ表示されるようにしています。
https://github.com/yhayashi30/lwc-custom-data-type-sample
Custom Data Typeを定義するためのdatatableButtonTypeというコンポーネントを作ります。コンポーネントのフォルダでは以下のようにdetailButton.htmlとrelatedListButton.htmlの2つのCustom Typeを定義します。
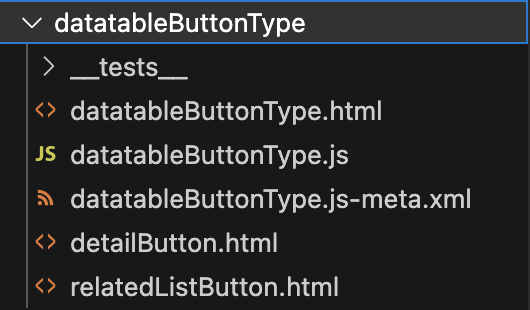
datatableButtonType.jsでは、LightningDatatableを継承してCutstom Typeの型とtemplate ファイルを特定しています。このサンプルでは、detailButton.htmlとrelatedListButton.htmlを使って2つのカスタムのボタンタイプを作っています。
typeAttributesでは、Array型でカスタムデータのtemplateへ必要なパラメータを渡すことができます。
import LightningDatatable from 'lightning/datatable';
import detailButton from './detailButton.html';
import relatedListButton from './relatedListButton.html';
export default class DatatableButtonType extends LightningDatatable {
static customTypes = {
detailButton: {
template: detailButton,
// Provide template data here if needed
typeAttributes: ['attrA', 'attrB'],
},
relatedListButton: {
template: relatedListButton,
// Provide template data here if needed
typeAttributes: ['attrA', 'attrB'],
}
//more custom types here
};
}
このサンプルでは、Custom Typeで表示する中身は別のコンポーネントとしています。valueでは、Datatableで表示している行のレコードIDがバインドされます。typeAttributes.attributeNameでは、Custom Typeで定義したパラメータをバインドします。
<template>
<c-datatable-related-list-Button
row-id={value}
attr-a={typeAttributes.attrA}
attr-b={typeAttributes.attrB}>
</c-datatable-related-list-Button>
</template>
サンプルでは、渡されたレコードIDで取引先に関連するケースの件数を確認して、1件以上であればボタンを表示するようにしております。
import { LightningElement, api, wire } from 'lwc';
import { getRelatedListCount } from 'lightning/uiRelatedListApi';
export default class DatatableRelatedListButton extends LightningElement {
@api rowId;
records;
error;
@api attrA;
@api attrB;
@wire(getRelatedListCount, {
parentRecordId: '$rowId',
relatedListId: 'Cases'
})listInfo({ error, data }) {
if (data) {
this.records = data.count > 0 ? true : false;
this.error = undefined;
} else if (error) {
this.error = error;
this.records = undefined;
}
}
fireRelatedListRow() {
const event = CustomEvent('relatedlistrow', {
composed: true,
bubbles: true,
detail: {
rowId: this.rowId,
},
});
this.dispatchEvent(event);
}
}
<template>
<template lwc:if={records}>
<div style="text-align: center;">
<lightning-button-icon
icon-name="utility:summarydetail"
onclick={fireRelatedListRow}>
</lightning-button-icon>
</div>
</template>
</template>
参考
Datatable
https://developer.salesforce.com/docs/component-library/bundle/lightning-datatable/documentation
Create a Custom Data Type
https://developer.salesforce.com/docs/component-library/documentation/en/lwc/lwc.data_table_custom_types